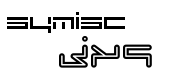
An Embeddable Scripting Engine |
Tweet |
Follow @jx9_engine |
JX9 C/C++ API Reference - List Of Functions.
This is a list of all functions and methods operating on the objects and using and/or returning constants. There are many functions, but most applications only use a handful.
For an introduction to the JX9 C/C++ API, please refer to the API intro page.
Engine Handling Interfaces |
Compile Interfaces |
Virtual Machine Handling Interfaces |
In-process Extending Interfaces |
Foreign Function Parameter Values |
Setting The Result Of A Foreign Function |
Call Context handling Interfaces |
Call Context Memory Management Interfaces |
Dynamically Typed Value Object Management Interfaces |
Global Library Management Interfaces |
|
|
On Demand Dynamically Typed Value Object allocation interfaces |
JSON Arrays/Objects Management Interfaces |
jx9_init
int jx9_init(jx9 **ppEngine);
Allocate and initialize a new JX9 engine instance.
Description
This routine create, initialize and returns a new JX9 engine instance. This is often the first JX9 API call that an application makes and is a prerequisite in order to compile JX9 code using one of the compile interfaces.
Parameters
ppEngine |
OUT: A pointer to a freshly initialized JX9 engine is stored here. When something goes wrong, this pointer is left pointing to NULL. |
Return value
JX9_OK is returned on success. Any other return value typically JX9_NOMEM (Out of memory) indicates failure and the caller must discard the ppEngine pointer.
jx9_config
int jx9_config(jx9 *pEngine,int nConfigOp,...);
Configure a JX9 engine instance.
Description
This routine is used to configure a working JX9 engine instance created by a prior successful call to jx9_init().
The second argument to jx9_config() is an integer configuration option that determines what property of JX9 is to be configured. Subsequent arguments vary depending on the configuration option in the second argument. Here is the list of allowed verbs
Configuration Verb |
Expected Arguments |
Description |
JX9_CONFIG_ERR_LOG |
Two Arguments: const char **pzPtr, int *pLen;
|
When something goes wrong while compiling a JX9 script due to an erroneous JX9 code, the engine error log is redirected to an internal buffer. This option is used to extract that log. The first argument is a pointer address. This pointer will be automatically set by the engine to point to the error log buffer which is a null terminated string. The second argument this option takes is an optional pointer to an integer. When set (i.e. not null), the integer value will hold the length of the error log buffer. Example: const char *zErrLog; int nLen; /* Extract error log */ jx9_config( JX9_CONFIG_ERR_LOG, &zErrLog, /* First arg*/ &nLen /* Second arg */ ); if( nLen > 0 ){ puts(zErrLog); /* Output*/ } |
JX9_CONFIG_ERR_ABORT |
No Arguments |
This configuration verb is reserved for future use and is a no-op in the current release of the JX9 engine. |
Parameters
pEngine |
A pointer to a correctly initialized JX9 engine. |
nConfigOp |
An integer configuration option that determines what property of the JX9 engine is to be configured. |
Return value
JX9_OK is returned on success. Any other return value typically JX9_CORRUPT (Unknown configuration verb) indicates failure.
jx9_release
int jx9_release(jx9 *pEngine);
Destroy a JX9 engine instance.
Description
This routine destroy a working JX9 engine instance created by a prior successful call to jx9_init(). Every JX9 engine must be destroyed using a call to this routine in order to avoid memory leaks .
Parameters
pEngine |
A pointer to a correctly initialized JX9 engine. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
jx9_compile
int jx9_compile(
jx9 *pEngine,
const char *zSource,
int nLen,
jx9_vm **ppOutVm
);
Compile a JX9 program to JX9 bytecodes.
Description
To execute JX9 code, it must first be compiled into a bytecode program using one of the compile routines.
This routine takes as its input a JX9 program to compile and produce JX9 bytecodes represented by an opaque pointer to the jx9_vm structure.
This API does not actually evaluate the JX9 code. It merely prepares the JX9 code for later execution.
On successful compilation, the compiled program is stored in the ppOutVm pointer (Fourth parameter) and the program is ready for execution using jx9_vm_exec(). When something goes wrong while compiling the JX9 script due to a compile-time error, the caller must discard the ppOutVm pointer and fix its erroneous JX9 code. The compile-time error log can be extracted using the jx9_config() interface with a configuration verb set to:
Parameters
pEngine |
A pointer to a correctly initialized JX9 engine. |
zSource |
JX9 program to compile. |
nLen |
Source length. If the nLen argument is less than zero, then zSource is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zSource. |
ppOutVm |
Out: On successful compilation, *ppOutVm is left pointing to the compiled JX9 program represented by an opaque pointer to the jx9_vm structure. It's safe now to call one of the VM management interfaces such as jx9_vm_config() and the compiled program can be executed using jx9_vm_exec(). Otherwise *ppOutVM is left pointing to NULL. |
Return value
JX9_OK is returned on success. Any other return value indicates failure such as:
JX9_COMPILE_ERR is returned when compile-time errors occurs. That is, the caller must fix its erroneous JX9 code and call the compile interface again.
Note that the compile-time error log can be extracted using the jx9_config() interface with a configuration verb set to:
JX9_VM_ERR is returned when an error occur while initializing the virtual machine. This kind of error is typically due to a memory failure problem. But remember this is a very unlikely scenario on modern hardware even on modern embedded system.
Example
Download this C file for a smart introduction to the compile interfaces.
jx9_compile_file
int jx9_compile_file(
jx9 *pEngine,
const char *zFilePath,
jx9_vm **ppOutVm
);
Compile a JX9 File to JX9 bytecodes.
Description
To execute JX9 code, it must first be compiled into a bytecode program using one of the compile routines.
This routine takes as its input a JX9 file to compile and produce JX9 bytecodes represented by an opaque pointer to the jx9_vm structure.
This API does not actually evaluate the JX9 code. It merely prepares the JX9 code for later execution.
On successful compilation, the compiled program is stored in the ppOutVm pointer (Third parameter) and the program is ready for execution using jx9_vm_exec(). When something goes wrong while compiling the JX9 program due to a compile-time error, the caller must discard the ppOutVm pointer and fix its erroneous JX9 code. The compile-time error log can be extracted using the jx9_config() interface with a configuration verb set to:
Parameters
pEngine |
A pointer to a correctly initialized JX9 engine. |
zFilePath |
Relative or full path to the JX9 file to compile. Note that this function depends on the
xmmap() routine of the underlying VFS. In
other words it tries to get a read-only memory view of the whole file.
Windows and UNIX users don't have to worry about these details since
JX9 come with a built-in VFS that handle all the stuff. But others
platforms must register their own VFS (Virtual File System) that
implements the xmmap() routine. Otherwise this function fail and JX9_IO_ERR is returned. |
ppOutVm |
Out: On a successful compilation, *ppOutVm is left pointing to the compiled JX9 program represented by an opaque pointer to the jx9_vm structure. It's safe now to call one of the VM management interfaces such as jx9_vm_config() and the compiled program can be executed using jx9_vm_exec(). Otherwise *ppOutVM is left pointing to NULL. |
Return value
JX9_OK is returned on success. Any other return value indicates failure such as:
JX9_COMPILE_ERR is returned when compile-time errors occurs. That is, the caller must fix its erroneous JX9 code and call the compile interface again.
Note that the compile-time error log can be extracted using the jx9_config() interface with a configuration verb set to:
JX9_VM_ERR is returned when an error occur while initializing the virtual machine. This kind of error is typically due to a memory failure problem. But remember this a very unlikely scenario on modern hardware even on modern embedded system.
JX9_IO_ERR is returned when IO errors occurs (i.e. permission error, nonexistant file, etc.) or the underlying VFS does not implement the xmmap() method which is not the case when the built-in VFS is used (default case).
Example
Download this C file for a smart introduction to the compile interfaces.
jx9_vm_config
int jx9_vm_config(jx9_vm *pVm,int iConfigOp,...);
Configure a JX9 Virtual machine.
Description
This routine is used to configure a JX9 virtual machine obtained by a prior successful call to one of the compile interface such as jx9_compile() or jx9_compile_file().
The second argument to jx9_vm_config() is an integer configuration option that determines what property of the JX9 virtual machine is to be configured. Subsequent arguments vary depending on the configuration option in the second argument. There are many verbs but the most important are JX9_VM_CONFIG_OUTPUT, JX9_VM_CONFIG_CREATE_VAR, JX9_VM_CONFIG_HTTP_REQUEST and JX9_VM_CONFIG_ARGV_ENTRY. Here is the list of allowed verbs
Configuration Verb |
Expected Arguments |
Description |
Two Arguments: int (*xConsumer)( const void *pOutput, unsigned int nLen, void *pUserData ), void *pUserData;
|
This option is used to install a VM output consumer callback. That is, an user defined function responsible of consuming the VM output such as redirecting it (The VM output) to STDOUT or sending it back to the connected peer. This option accepts two arguments. The first argument is a pointer to the user defined function responsible of consuming the VM output. The callback must accept three arguments. The first argument is a pointer to the VM generated output that the callback body can safely cast to a string (const char *). Note that if the pointer is cast-ed to a string, keep in mind that is not null terminated. The second argument is the VM output length in bytes. The virtual machine guarantee this number is always greater than zero. The last argument is a copy of the pointer (callback private data) passed as the second argument to the this option. When done, the callback must return JX9_OK. But if for some reason the callback wishes to abort processing and thus to stop program execution, it must return JX9_ABORT instead of JX9_OK. Note that the virtual machine will invoke this callback each time it have something to output. Refer to the following article for an introduction and some working example of VM output consumer callbacks. The last argument this option takes is an arbitrary user pointer forwarded verbatim by the engine to the callback as its last argument.
|
|
JX9_VM_CONFIG_IMPORT_PATH |
One Argument: const char *zPath; |
This option add a path to the import search directories. That is, if a local file is included from the running script using the include or import JX9 constructs, then the given path is used as one of the search directory. This option takes a single argument which is a pointer to a NULL terminated string holding the search path. Example: /* Import from local/include*/ jx9_vm_config( pVm, JX9_VM_CONFIG_IMPORT_PATH, "/usr/local/include" );
|
No Arguments |
This option takes no arguments and if set, all run-time errors such as call to an undefined function, instantiation of an undefined class and so on are reported in the VM output. |
|
JX9_VM_CONFIG_RECURSION_DEPTH |
One Argument: int nMaxDepth
|
This option is used to set a recursion limit to the running script. That is, a function may not call itself (recurse) more than this limit. If this limit is reached then the virtual machine abort the call and null is returned to the caller instead of the function return value. Note that passing this limit will not stop program execution, simply a null value is returned instead. The default limit (depending on the host environment) is adequate for most situations (JX9 is smart enough to peek the appropriate value) and thus we recommend the users to not set a limit of their own unless they know what they are doing. This option accept a single argument which is an integer between 0 and 1024 specifying the desired recursion limit. |
JX9_VM_OUTPUT_LENGTH |
One Argument: unsigned int *pLength
|
This option takes a single argument which is the address of an unsigned integer. The value of this integer will hold the total number of bytes that have been outputted by the Virtual Machine during program execution. |
Two Arguments: const char zVarname, jx9_value *pValue |
This option is used to register a foreign variable within the running JX9 script. This option is useful if you want to pass information from the outside environment to the target JX9 script such as your application name, version, copyright or some environments variables and so forth. This option accepts two arguments. The first argument is a pointer to a null terminated string holding the name (without the dollar sign) of the foreign variable to be installed such as APP_NAME, APP_VERSION, etc. and the second argument is a pointer to a jx9_value obtained by a prior successful call to jx9_new_scalar() or jx9_new_array(). Use the following interfaces to populate the jx9_value with the desired value: After installing the foreign variable, it is recommended that your release its associated value using the jx9_release_value() interface since JX9 will make a private copy of the installed value. Download and compile this C file for an usage example on how data is shared between the host application and the running JX9 script. |
|
Two Arguments: const char *zRequest, int nByte |
This option is used to pass HTTP request information to the target JX9 script. JX9 is shipped with a powerful HTTP request parser and a query decoder for GET, POST and PUT methods. All you have to do is read the raw HTTP request using the standard system call such read() or recv() and pass a pointer to the buffer used for reading the request to this interface and let JX9 parse the request, decode the queries and fill the appropriate JSON objects such as $_GET, $_POST, $_REQUEST, $_SERVER, etc. This option must be called prior to program execution so that the JX9 engine can populate the appropriate JSON objects. This option accept two arguments. The first argument is a pointer to the raw HTP request just read and the second argument is the HTTP request length (data just read) in bytes. You can refer to the following article for an introduction on how to integrate JX9 in web applications (including servers) that deal with the HTTP protocol.
|
|
JX9_VM_CONFIG_SERVER_ATTR |
Three Arguments: const char *zKey, const char *zValue, int nLen
|
This option is used to manually populate the $_SERVER predefined JSON object which hold sever environments entries. This option takes three arguments. The first argument is a pointer to a null terminated string holding the entry key, the second argument is a pointer to a string holding the entry value (This string may not be null terminated). The third argument is the zValue length in bytes. If the nLen argument is less than zero, then zValue is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zValue. Example: jx9_vm_config( pVm, JX9_VM_CONFIG_SERVER_ATTR, "HTTP_CONNECTION", "Keep-Alive", -1 ); jx9_vm_config( pVm, JX9_VM_CONFIG_SERVER_ATTR, "HTTP_HOST", "localhost", 9 ); Now $_SERVER.HTTP_CONNECTION; //Keep-Alive and $_SERVER.HTTP_HOST; //localhost |
JX9_VM_CONFIG_ENV_ATTR |
Three Arguments: const char *zKey, const char *zValue, int nLen |
This option is used to manually populate the $_ENV predefined JSON object which hold environments variable. This option takes three arguments. The first argument is a pointer to a null terminated string holding the entry key, the second argument is a pointer to a string holding the entry value (This string may not be null terminated). The third argument is the zValue length in bytes. If the nLen argument is less than zero, then zValue is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zValue. |
JX9_VM_CONFIG_EXEC_VALUE |
One Argument: jx9_value **ppValue |
This option takes one
argument which is the address of a pointer to a jx9_value. This will point to the return value of the running
script (usually
NULL). |
JX9_VM_CONFIG_IO_STREAM |
One Argument: const jx9_io_stream *pStream
|
This option takes a single argument which is a pointer to an instance of the jx9_io_stream structure. A pointer to this instance will be stored in an internal container of the underlying virtual machine so that the scheme associated with this IO stream (I.e: file://, http://, etc.) can be invoked from fopen(), fread(), frwite() calls. Note that JX9 will not make a private copy of the content of the jx9_io_stream structure which mean that this instance must be available during the life of the library. You can refer to this page for an introduction to the JX9 IO streams. |
JX9_VM_CONFIG_ARGV_ENTRY |
One Argument: const char *zArgValue; |
This option is used to populate the $argv predefined JSON object. The only argument this option takes is a pointer to a null terminated string holding the script argument value to insert. Example: jx9_vm_config( pVm, JX9_VM_CONFIG_ARGV_ENTRY, "arg1" ); jx9_vm_config( pVm, JX9_VM_CONFIG_ARGV_ENTRY, "arg2" ); Now, $argv[0] value is “arg1” and $argv[1] value is “arg2”
|
Two Arguments: const void **ppOut, unsigned int *pLen; |
If the host application did not install a VM output consumer callback (This can be done via JX9_VM_CONFIG_OUTPUT), then the Virtual Machine will automatically redirect its output to an internal buffer. This option can be used to extract that buffer. The first argument this option takes is a pointer address. This pointer will be automatically set by the VM to point to the internal output buffer which is not null terminated. The second argument is the address of an unsigned integer. This integer will hold the length of the VM output buffer. This option must be called after successful call to jx9_vm_exec() and before jx9_vm_reset() or jx9_vm_release() Example: /* Execute the script */ rc = jx9_vm_exec(pVm,0); if( rc != JX9_OK ){ Fatal("VM execution error"); } { const void *pOut; unsigned int nLen; /* Extract the output */ jx9_vm_config( pVm, JX9_VM_CONFIG_EXTRACT_OUTPUT, &pOut, &nLen ); if( nLen > 0 ){ /* Redirect to STDOUT */ printf("%.*s", (int)nLen, (const char *)pOut /* Not NULL terminated */ ); } } jx9_vm_reset(pVm); Note that for performance reason it is preferable to install a VM output consumer callback via (JX9_VM_CONFIG_OUTPUT) rather than waiting for the VM to finish executing and extracting the output. |
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
nConfigOp |
An integer configuration option that determines what property of the JX9 virtual machine is to be configured. |
Return value
JX9_OK is returned on success. Any other return value typically JX9_CORRUPT (Unknown configuration verb) indicates failure.
jx9_vm_exec
int jx9_vm_exec(jx9_vm *pVm,int *pExitStatus);
Execute a compiled JX9 program.
Description
This routine is used to execute JX9 bytecode program resulting from successful compilation of the target JX9 script using one of the compile interfaces such as jx9_compile() or jx9_compile_file(). If no output consumer callback were installed, then the virtual machine will automatically redirect its output to an internal buffer. The caller can point to that buffer later when the VM have finished executing the program via a call to jx9_vm_config() with a configuration verb set to JX9_VM_CONFIG_EXTRACT_OUTPUT. But remember for performance reason it is preferable to install a VM output consumer callback rather than waiting for the VM to finish executing and extracting the output.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
pExitStaus |
Optional: If the caller is interested in extracting the exit status of the program then this integer will hold the exit status number (typically zero). Otherwise pass a NULL pointer. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
Example
Download this C file for a smart introduction to this interface.
jx9_vm_extract_variable
jx9_value * jx9_vm_extract_variable(jx9_vm *pVm,const char *zVarname);
Description
This routine is used to extract the content of some variable declared inside your JX9 script which is a very useful way to share information between the host application and the target JX9 script.
After successful return from this routine, you can use the following interface to extract the raw content of the variable:
If you want to modify the content of the extracted variable, use the following interfaces:
This routine must be called after execution of the target JX9 code. Otherwise, NULL is returned.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
zVarname |
A pointer to a null terminated string holding the name of the variable to be extracted. |
Return value
Variable content is returned on success. NULL is returned on failure (i.e. variable not declared).
Example
Download this C file for a smart introduction to this interface.
jx9_vm_reset
int jx9_vm_reset(jx9_vm *pVm);
Reset a JX9 Virtual machine to its initial state.
Description
This routine is used to reset the Virtual Machine to its initial state so that the caller can safely recall jx9_vm_exec() and execute the compiled program again.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
Return value
JX9_OK is returned on success. Any other return value typically JX9_CORRUPT indicates failure.
jx9_vm_release
int jx9_vm_release(jx9_vm *pVm);
Destroy a JX9 Virtual machine.
Description
This routine destroy a working JX9 virtual machine. Every virtual machine must be destroyed using a call to this routine in order to avoid memory leaks.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
Return value
JX9_OK is returned on success. Any other return value typically JX9_CORRUPT indicates failure.
jx9_vm_dump_v2
int jx9_vm_dump_v2(
jx9_vm *pVm,
int (*xConsumer)(const void *pDump,unsigned int nLen,void *pUserData),
void *pUserData
);
Dump JX9 Virtual machine instructions.
Description
This routine is used to dump JX9 bytecode instructions to a human readable format. The dump is redirected to the given consumer callback which is responsible of consuming the generated dump perhaps redirecting it to its standard output (STDOUT).
Parameter
pVm
|
A pointer to a JX9 Virtual Machine. |
int (*xConsumer)(const void *,unsigned int,void *) |
Dump consumer callback. The callback must accept three parameters. The first parameter is the generated dump which is not null terminated. The second parameter is the dump length in bytes (The engine guarantee this number is always greater than zero) and the last parameter is the callback private data (arbitrary user pointer forwarded verbatim by the engine). The callback must return JX9_OK. Otherwise the dump generation is aborted immediately. |
pUserData |
An arbitrary user pointer forwarded verbatim by the engine to the callback as its third argument. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
jx9_create_function
int jx9_create_function(
jx9_vm *pVm,
const char *zName,
int (*xFunc)(jx9_context *pCtx,int argc,jx9_value **argv),
void *pUserData
);
Install a foreign function and invoke it from your JX9 code.
Description
This interface known as "foreign function creation routine" is used to add JX9 functions or to redefine the behavior of existing JX9 functions. After successful call to this interface, the installed function is available immediately and can be called from the target JX9 code.
The first parameter is the virtual machine to which the JX9 function is to be added. If an application uses more than one virtual machine then application-defined JX9 functions must be added to each virtual machine separately.
The second parameter is a pointer to a null terminated string holding the name of the JX9 function to be created or redefined. A valid function name starts with a letter or underscore, followed by any number of letters, numbers, or underscores. Also note that functions names under JX9 are case sensitive.
The third and most important parameter is a pointer to C-language function that implement the JX9 function. This function must accept three parameters. The first parameter is a pointer to a jx9_context structure. This is the context in which the foreign function executes. In other words, this parameter is the intermediate between the foreign function and the underlying virtual machine.
The application-defined foreign function implementation will pass this pointer through into calls to dozens of interfaces, these includes:
jx9_context_output(), jx9_context_throw_error(), jx9_context_new_scalar(), jx9_context_user_data(), jx9_context_alloc_chunk() and many more.
The computation result (i.e: the return value) of the foreign function can be set via one of these interfaces:
If no call is made to one of these interfaces, then a NULL return value is assumed.
The second parameter xFunc() takes is the total number of arguments passed to the foreign function. If the total number of arguments is not the expected one, the foreign functions can throw an error via jx9_context_throw_error() as follow:
jx9_context_throw_error(pCtx,JX9_CTX_WARNING,"Unexpected number of arguments");
The last parameter xFunc() takes is an array of pointers to jx9_value which represents function arguments. The implementation of the foreign functions can extract their contents via one of these interfaces:
The xFunc() implementation must return JX9_OK on success. But if the callbacks wishes to abort processing (to stop program execution) and thus to emulate the exit() or die() JX9 constructs, it must return JX9_ABORT instead.
The fourth and last parameter to jx9_create_function() is an arbitrary pointer. The implementation of the function can gain access to this pointer using jx9_context_user_data(). Also note that foreign functions can store an arbitrary number of auxiliary private data in a stack-able manner via jx9_context_push_aux_data().
Built-in functions may be overloaded by new application-defined functions. Note that JX9 come with more than 303 built-in functions installed using exactly this interface.
This function must be called before program execution via jx9_vm_exec().
You can refer to the following guide for an introductory course to this interface and the foreign function mechanism in general.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
zName |
A pointer to a null terminated string holding the name of the foreign function. |
xFunc |
A pointer to a C function performing the desired computation. |
pUserData |
Arbitrary user pointer which can be extracted later in the implementation of the foreign function via a call to jx9_context_user_data(). |
Return value
JX9_OK is returned on success. Any other return value typically (JX9_NOMEM) indicates failure.
Example
Download this C file for a smart introduction to this interface.
jx9_delete_function
int jx9_delete_function(jx9_vm *pVm,const char *zName);
Delete a foreign function.
Description
This routine deletes an installed foreign function. That is, any future invocation of the function will throw a call to an undefined function error.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
zName |
A pointer to a null terminated string holding the name of the foreign function to be deleted. |
Return value
JX9_OK is returned on success. Any other return value indicates failure (no such function).
jx9_create_constant
int jx9_create_constant(
jx9_vm *pVm,
const char *zName,
void (*xExpand)(jx9_value *pValue,void *pUserData),
void *pUserData
);
Constant Expansion Mechanism: Expand a constant to the desired value via user installable callbacks.
Description
This routine is used to register constants which are expanded to the desired value when invoked later from the running JX9 script.
The constant expansion mechanism under JX9 is extremely powerful yet simple and work as follows:
Each registered constant have a C procedure associated with it. This procedure known as the constant expansion callback is responsible of expanding the invoked constant to the desired value. For example the C procedure associated with the “__PI__” constant expands to 3.14 (the value of PI), the “__OS__” constant expands to the name of the host Operating System (Windows, Linux) and so on.
The third and most important parameter this function takes is an application-defined procedure responsible of expanding the constant to the desired value. This procedure must accept two arguments. The first argument is a pointer to a jx9_value that the procedure must fill with the desired value for example 3.14 (value of PI) is stored in this pointer. Use the following interfaces to populate the jx9_value with the desired value:
The second argument is a copy of the fourth argument to this function which is forwarded verbatim by engine.
Built-in constants may be overloaded by new application-defined constants. Note that JX9 come with more than 139 built-in constants installed using exactly this interface.
A valid constant name starts with a letter or underscore, followed by any number of letters, numbers or underscores. Also note that constants names are case-sensitive.
This function must be called before program execution via jx9_vm_exec().
You can refer to the following guide for an introductory course to this interface and the constant expansion mechanism in general.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
zName |
A pointer to a null terminated string holding the name of the constant for example __PI__, __OS__, JX9_EOL. |
xExpand |
Host-application defined procedure responsible of expanding the constant to the desired value. |
pUserData |
Arbitrary user pointer which is forwarded verbatim by the engine to the callback as its second argument. |
Return value
JX9_OK is returned on success. Any other return value typically (JX9_NOMEM) indicates failure.
Example
Download this C file for a smart introduction to this interface.
jx9_delete_constant
int jx9_delete_constant(jx9_vm *pVm,const char *zName);
Delete a constant.
Description
This routine remove an installed constant from the constants table.
Parameters
pVm |
A pointer to a JX9 Virtual Machine. |
zName |
A pointer to a null terminated string holding the name of the constant to be deleted. |
Return value
JX9_OK is returned on success. Any other return value indicates failure (no such constant).
Foreign Function Parameter Values
int jx9_value_to_int(jx9_value *pValue);
int jx9_value_to_bool(jx9_value *pValue);
jx9_int64 jx9_value_to_int64(jx9_value *pValue);
double jx9_value_to_double(jx9_value *pValue);
const char * jx9_value_to_string(jx9_value *pValue,int *pLen);
void * jx9_value_to_resource(jx9_value *pValue);
int jx9_value_compare(jx9_value *pLeft,jx9_value *pRight,int bStrict);
Obtaining foreign function parameter values.
Description
The C-language implementation of JX9 functions uses this set of interface routines to access the parameter values on the function. The xFunc parameter to jx9_create_function() define callbacks that implement the JX9 functions. The 3rd parameter to these callbacks is an array of pointers to jx9_value objects. There is one jx9_value object for each parameter to the foreign JX9 function. These routines are used to extract values from the jx9_value objects. Please note that if the jx9_value is not of the expected type, an automatic cast will be performed.
The
jx9_value_to_string() interface takes an optional parameter
(int *pLen) which is set (if not NULL) to the string
length. Also note, this function return a null terminated string and
does never return the NULL pointer.
Please pay particular attention to the fact that the pointer returned from jx9_value_to_string() can be invalidated by subsequent call to jx9_value_to_int(), jx9_value_to_bool(), jx9_value_to_int64() or jx9_value_to_double().
The jx9_value_to_resource() interface will return NULL if the given jx9_value is not of type resource.
The jx9_value_compare() interface is used to compare two jx9_value's. The return value will be 0 (zero) if the values are equals, >0 if the left (first argument) value is greater than the right (second argument) and <0 if the left (first argument) value is less than the right (second argument).
These routines must be called from the same thread as the foreign function that supplied the jx9_value parameters.
Parameters
pValue |
A pointer to a jx9_value. |
Return value
Value of the given parameter.
Example
Download this C file for a smart introduction to these interfaces.
Dynamically Typed Value Object Management Interfaces
int jx9_value_is_int(jx9_value *pVal);
int jx9_value_is_float(jx9_value *pVal);
int jx9_value_is_bool(jx9_value *pVal);
int jx9_value_is_string(jx9_value *pVal);
int jx9_value_is_null(jx9_value *pVal);
int jx9_value_is_numeric(jx9_value *pVal);
int jx9_value_is_callable(jx9_value *pVal);
int jx9_value_is_scalar(jx9_value *pVal);
int jx9_value_is_json_array(jx9_value *pVal);
int jx9_value_is_json_object(jx9_value *pVal);
int jx9_value_is_resource(jx9_value *pVal);
int jx9_value_is_empty(jx9_value *pVal);
Check if the given jx9_value is of the expected type.
Description
As their name suggests, these routines returns TRUE if the given jx9_value is of the expected type as requested by the caller. For example jx9_value_is_int() return TRUE only if the given jx9_value is of type integer, jx9_value_is_string() return TRUE only if the given jx9_value is of type string and so forth.
Note that these routines does not perform any automatic conversion when checking jx9_value type. If such behavior is wanted, please use jx9_value_to_string(), jx9_value_to_int(), etc. instead.
These routines are useful inside foreign functions to make sure that the given arguments are of the expected type.
The jx9_value_is_callable() interface does not check for a type but instead check if the given jx9_value is a callback. That is, a function that can be called from the target JX9 code.
Parameters
pValue |
A pointer to a jx9_value. |
Return value
These routines returns 1 (TRUE) if the given jx9_value is of the expected type. 0 (zero) (FALSE) otherwise.
Example
Download this C file for a smart introduction to these interfaces.
Populating Dynamically Typed Value Object
int jx9_value_int(jx9_value *pVal,int iValue);
int jx9_value_int64(jx9_value *pVal,jx9_int64 iValue);
int jx9_value_bool(jx9_value *pVal,int iBool);
int jx9_value_null(jx9_value *pVal);
int jx9_value_double(jx9_value *pVal,double Value);
int jx9_value_string(jx9_value *pVal,const char *zString,int nLen);
int jx9_value_string_format(jx9_value *pVal,const char *zFormat,...);
int jx9_value_reset_string_cursor(jx9_value *pVal);
int jx9_value_resource(jx9_value *pVal,void *pUserData);
Populate a jx9_value with the desired value.
Description
These routines are used to populate a freshly created jx9_value obtained by a prior successful call to jx9_context_new_scalar() or from a callback responsible of expanding constant value.
The jx9_value_int() interface sets the jx9_value to the integer type where the value is specified by its 2nd argument.
The jx9_value_int64() interface sets the jx9_value to the 64-bit integer type where the value is specified by its 2nd argument.
The jx9_value_double() interface sets the jx9_value to the double type where the value is specified by its 2nd argument.
The jx9_value_bool() interface sets the jx9_value to the Boolean type where the value (Only zero is assumed to be FALSE) is specified by its 2nd argument.
The jx9_value_null() interface sets the jx9_value to the NULL type.
The jx9_value_resource() interface sets the jx9_value to the resource type where the value is specified by its 2nd argument which is an arbitrary user pointer that can be extracted later using jx9_value_to_resource().
The jx9_value_string() interface sets the jx9_value to the string type where the string content is specified by the 2nd argument.
Note that jx9_value_string() accepts a third argument which is the length of the string to append. If the nLen argument is less than zero, then zString is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zString.
jx9_value_string_format() is a work-alike of the "printf()" family of functions from the standard C library which is used to append a formatted string.
The jx9_value_string() and jx9_value_string_format() interfaces write their result to an internal buffer that grow automatically by successive calls to one of them (An append operation). That is, previously written data is not erased by the new call. If such behavior is wanted, call jx9_value_reset_string_cursor() to reset the internal buffer.
Example
jx9_value_string(pValue,"Welcome, current time is: ",-1);
jx9_value_string_format(pValue,"%02d:%02d:%02d",14,12,59);
The final result if extracted by jx9_value_to_string() would look like this:
“Welcome, current time is: 14:12:59”
These routines are very useful when implementing foreign functions that need to populate JSON arrays or objects values.
If these routines are called from within the different thread than the one containing the application-defined function that received the jx9_value pointer, the results are undefined.
Parameters
pValue |
A pointer to a jx9_value to populate. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
Example
Download this C file for a smart introduction to these interfaces.
Setting The Result Of A Foreign Function
int jx9_result_int(jx9_context *pCtx,int iValue);
int jx9_result_int64(jx9_context *pCtx,jx9_int64 iValue);
int jx9_result_bool(jx9_context *pCtx,int iBool);
int jx9_result_double(jx9_context *pCtx,double Value);
int jx9_result_null(jx9_context *pCtx);
int jx9_result_string(jx9_context *pCtx,const char *zString,int nLen);
int jx9_result_string_format(jx9_context *pCtx,const char *zFormat,...);
int jx9_result_value(jx9_context *pCtx,jx9_value *pValue);
int jx9_result_resource(jx9_context *pCtx,void *pUserData);
Set the return value of a foreign function.
Description
These routines are used by the xFunc() callback that implement foreign JX9 functions to return their computation result. See jx9_create_function() for additional information.
These functions work very much like the jx9_value_* family of functions used to populate jx9 values.
The jx9_result_int() interface sets the result from an application-defined function to be an integer value specified by its 2nd argument.
The jx9_result_int64() interface sets the result from an application-defined function to be a 64-bit integer value specified by its 2nd argument.
The jx9_result_double() interface sets the result from an application-defined function to be a floating point value specified by its 2nd argument.
The jx9_result_bool() interface sets the result from an application-defined function to be a Boolean value (Only zero is assumed FALSE) specified by its 2nd argument.
The jx9_result_null() interface sets the return value of the application-defined function to be NULL (default return value).
The jx9_result_resource() interface sets the result from an application-defined function to be a resource value specified by its 2nd argument which is an arbitrary user pointer that can be extracted later using jx9_value_to_resource().
The jx9_result_value() interface sets the result from an application-defined function to be a copy of the jx9_value obtained by a prior successful call to jx9_context_new_scalar() or jx9_context_new_array(). This is how JSON arrays/Objects are returned (Download this C file for a working example).
The jx9_result_string() interface sets the result from an application-defined function to be a string value whose content is specified by the 2nd argument.
Note that jx9_result_string() accepts a third argument which is the length of the string to append. If the nLen argument is less than zero, then zString is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zString.
jx9_result_string_format() is a work-alike of the "printf()" family of functions from the standard C library which is used to append a formatted string.
Note that the printf() implementation in the JX9 engine is based on the one found in the SQLite3 source tree.
The jx9_result_string() and jx9_result_string_format() interfaces write their result to an internal buffer that grow automatically by successive calls to one of them (An append operation). That is, previously written data is not erased by the new call.
Example
jx9_result_string(pCtx,"Welcome, current time is: ",-1);
jx9_result_string_format(pCtx,"%02d:%02d:%02d",14,12,59);
The final return value of the foreign functions would look like this:
“Welcome, current time is: 14:12:59”
If these routines are called from within the different thread than the one containing the application-defined function that received the jx9_context pointer, the results are undefined.
You can refer to the following guide for an introductory course to this interface and the foreign function mechanism in general.
Parameters
pCtx |
Foreign function call context. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
Example
Download this C file for a smart introduction to these interfaces.
jx9_context_output
int jx9_context_output(jx9_context *pCtx,const char *zString,int nLen);
int jx9_context_output_format(jx9_context *pCtx,const char *zFormat,...);
Output a message.
Description
These routines when invoked redirects the desired message to the installed VM Output Consumer Callback. That is, these routines emulate the print JX9 language construct inside foreign functions.
Note that jx9_context_output() accepts a third argument which is the length of the string to output. If the nLen argument is less than zero, then zString is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zString.
jx9_context_output_format() is a work-alike of the "printf()" family of functions from the standard C library which is used to output a formatted string.
These routines accepts as their first arguments a pointer to a jx9_context which mean that they are designed to be invoked only from a foreign function.
These routines must be called from the same thread in which the application-defined function is running.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
Example
Download this C file for a smart introduction to these interfaces.
jx9_context_throw_error
int jx9_context_throw_error(jx9_context *pCtx,int iErr,const char *zErr);
int jx9_context_throw_error_format(jx9_context *pCtx,int iErr,const char *zFormat,...);
Throw an error message inside a foreign function.
Description
These
routines when invoked throw an error message to the installed VM
Output Consumer Callback.
Note
that the underlying Virtual
Machine
continues its execution normally when an error is thrown. If the
caller want to stop program execution and thus to
emulate the die
JX9 language construct inside a foreign function, simply return JX9_ABORT
instead of JX9_OK;
Note that jx9_context_throw_error() accepts a third argument which is the length of the string to output. If the nLen argument is less than zero, then zString is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zString.
jx9_context_throw_error_format() is a work-alike of the "printf()" family of functions from the standard C library which is used to output a formatted string.
These routines accepts as their first arguments a pointer to a jx9_context which mean that they are designed to be invoked only from a foreign function.
These routines must be called from the same thread in which the application-defined function is running.
Parameters
pCtx |
Foreign Function Call Context. |
iErr |
Error message severity level. This can be one of the following constants (Not a combination): JX9_CTX_ERR Call context error such as unexpected number of arguments, invalid types and so on. JX9_CTX_WARNING Call context warning. JX9_CTX_NOTICE Call context notice. |
Return value
JX9_OK is returned on success. Any other return value indicates failure.
Example
Download this C file for a smart introduction to these interfaces.
On demand jx9_value allocation
jx9_value * jx9_context_new_scalar(jx9_context *pCtx);
jx9_value * jx9_context_new_array(jx9_context *pCtx);
void jx9_context_release_value(jx9_context *pCtx,jx9_value *pValue);
jx9_value * jx9_new_scalar(jx9_vm *pVm);
jx9_value * jx9_new_array(jx9_vm *pVm);
int jx9_release_value(jx9_vm *pVm,jx9_value *pValue);
Allocate jx9_value on demand.
Description
These routine allocate jx9_value on demand, they are used mostly by foreign functions that populate and returns JSON arrays/objects.
jx9_context_new_scalar()
allocate a new scalar jx9_value
which is set to the null type by default. Use the following
interfaces to populate the jx9_value
with the desired value:
jx9_context_new_array()
allocate a new jx9_value of type array. The JSON array can be populated later
using one of these interfaces
jx9_array_add_elem() or jx9_array_add_strkey_elem(). This interface is unified for JSON arrays as well JSON objects.
Note that if you want to return the allocated jx9_value inside your foreign function, use jx9_result_value().
jx9_context_release_value() destroy a jx9_value obtained by a prior call to jx9_context_new_scalar() or jx9_context_new_array(). Note that this call is not necessary since the garbage collector subsystem will automatically destroy any allocated jx9_value as soon the foreign function have finished its execution.
These routines accepts as their first argument a pointer to a jx9_context which mean that they are designed to be invoked only from a foreign function.
jx9_new_scalar(), jx9_new_array() and jx9_release_value() follows the same semantics as jx9_context_new_scalar(), jx9_context_new_array() and jx9_context_release_value() except that they take as their first parameter a pointer to a jx9_vm instance. They are used for the creation of foreign variables that are registered later via jx9_vm_config() with a configuration verb set to JX9_VM_CONFIG_CREATE_VAR.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
jx9_context_new_scalar(), jx9_context_new_array(), jx9_new_scalar() and jx9_new_array() return a pointer to a freshly allocated jx9_value on success. Otherwise NULL is returned on failure (i.e: Out of memory).
Example
Download this C file for a smart introduction to these interfaces.
jx9_array_fetch
ph7_value * jx9_array_fetch(jx9_value *pArray,const char *zKey,int nByte);
Extract JSON array/object entry value.
Description
This routine is used to extract a JSON array/object entry value where zKey is its key. The jx9_value must be of type array or object obtained by a prior successful call to jx9_context_new_array() or passed as parameter to the foreign function. Otherwise this routine returns the NULL pointer in case the given jx9_value is not of type array/object.
If the target value is a JSON array (i.e. numerically indexed), you can pass numeric string keys such as “1”, “2”, “750”, etc. JX9 is smart enough to figure out that we are dealing with numeric keys.
If the given key does not refer to a valid entry then this routine return the NULL pointer. Otherwise the entry value is returned on success. This value can be manipulated or modified using one these interfaces and the change is immediately seen in the target JSON array/object:
Parameters
pArray |
jx9_value to query which must be of type array or object. |
zKey |
Lookup key. |
nByte |
zkey length. If this argument is less than zero, then zKey is read up to the first zero terminator. If nByte is non-negative, then it is the maximum number of bytes read from zKey. |
Return value
If the given key does not refer to a valid array/object entry or the given jx9_value is not of type array/object, then this function return the NULL pointer. Otherwise the entry value is returned on success.
Example
Download this C file for a smart introduction to the array handling interfaces.
jx9_array_walk
int jx9_array_walk(
jx9_value *pArray,
int (*xWalk)(jx9_value *pKey,jx9_value *pValue,void *pUserData),
void *pUserData
);
Iterate Over JSON array/object entries and invoke the supplied callback for each entry.
Description
This routine iterates over array/object entries and invokes the supplied walker callback for each entry. The first argument (pArray) must be of type array or object obtained by a prior successful call to jx9_context_new_array() or passed as parameter to the foreign function. Otherwise, this routine return immediately and the walk process could not be done.
The most interesting parameter this function takes is the walker callback which is an user defined function that is invoked for each array/object entry. The callback must accept three arguments. The first argument is the entry key and the second argument is the entry value which could be any type even others JSON arrays and objects and the third and last argument is a copy of the third argument to this function which is forwarded verbatim by engine.
The callback must return JX9_OK. Otherwise the walk process is aborted and this function return JX9_ABORT.
The key and entry value could be extracted inside the callback using these interfaces:
Note that the key and its value are passed to the callback by copy. That is, any subsequent changes to their types or values is not reflected. If you want to modify the entry value use jx9_array_fetch() instead.
Parameters
pArray |
jx9_value to walk which must be of type array/object. |
xWalk |
Walker callback that is invoked for each array entry. If the callback wishes to abort the walk process, it must return a value different from JX9_OK such as JX9_ABORT. |
pUserDara |
Arbitrary user pointer which is forwarded verbatim by the engine to the walker callback as its third argument. |
Return value
This function return JX9_OK on success. Any other return value indicates failure.
Example
Download this C file for a smart introduction to the array handling interfaces.
jx9_array_add_elem
int jx9_array_add_elem(jx9_value *pArray,jx9_value *pKey,jx9_value *pValue);
int jx9_array_add_strkey_elem(jx9_value *pArray,const char *zKey,jx9_value *pValue);
Populate JSON array/object values.
Description
These routines populates JSON array/object values. The given jx9_value (first argument) must be of type array/object obtained by a prior successful call to jx9_context_new_array() or jx9_new_array(). Otherwise these routines return immediately with an error code.
The second argument to jx9_array_add_elem() is a scalar jx9_value obtained by a prior successful call to jx9_context_new_scalar(), jx9_new_array() or passed as parameter to the foreign function. Note that if you want an automatically assigned key (usually a numeric number), simply pass a NULL pointer.
The third argument to jx9_array_add_elem() is a jx9_value of any type obtained by a prior successful call jx9_context_new_scalar(), jx9_new_scalar(), jx9_context_new_array(), jx9_new_array() or passed as parameter to the foreign function. This is is the entry value that could be extracted later using jx9_array_fetch() or from your JX9 code using the standard array access operator ($obj.key). Note that if you want a null entry value, simply pass a NULL pointer.
jx9_array_add_strkey_elem() is a simple wrapper around jx9_array_add_elem().
Note that the three interfaces listed above will make their own copy of the key and the value so it is safe to use the same jx9_value for other insertions.
Parameters
pArray |
jx9_value to populate which must be of type array/object. |
Return value
JX9_OK is returned on successful insertion. Any other return value typically (JX9_CORRUPT or JX9_NOMEM) indicates failure.
Example
Download this C file for a smart introduction to the array handling interfaces.
jx9_array_count
unsigned int jx9_array_count(jx9_value *pArray);
Return the total number of inserted entries in a JSON array/object.
Description
This routine return the total number of inserted entries in a given array/object. The jx9_value must be of type array/object obtained by a prior successful call to jx9_context_new_array() or passed as parameter to the foreign function.
Call jx9_value_is_json_array() or jx9_value_is_json_object() to check if the jx9_value is of the expected type.
Parameters
pArray |
jx9_value to query which must be of type array/object. |
Return value
This function return the total number of inserted entries on success. 0 (zero) is returned if the array/object is empty or the given jx9_value is not of type array (a scalar value such as an integer, Boolean, double, etc.).
Example
Download this C file for a smart introduction to the array handling interfaces.
Call Context Memory Management Interfaces
void * jx9_context_alloc_chunk(jx9_context *pCtx,unsigned int nByte,int ZeroChunk,int AutoRelease);
void * jx9_context_realloc_chunk(jx9_context *pCtx,void *pChunk,unsigned int nByte);
void jx9_context_free_chunk(jx9_context *pCtx,void *pChunk);
Memory Allocation Routines
Description
As their names suggests these interfaces allocate/re-allocate/free memory using the JX9 memory backend subsystem.
These interfaces accepts as their first argument a pointer to a jx9_context which mean that they are designed to be invoked only from a foreign function.
The jx9_context_alloc_chunk() routine returns a pointer to a block of memory at least nByte bytes in length, where nByte is the second parameter.
The last parameter jx9_context_alloc_chunk()
takes is a Boolean flag when set to TRUE (any value different
from zero) then the allocated chunk is automatically released upon the
foreign function have finished its execution. That is, there is no
need to manually call jx9_context_free_chunk().
The jx9_context_realloc_chunk() interface attempts to resize a prior memory allocation to be at least nByte bytes, where nByte is the third parameter. The memory allocation to be resized is the second parameter. If the second parameter to jx9_context_realloc_chunk() is a NULL pointer then its behavior is identical to calling jx9_context_alloc_chunk(nByte) where nByte is the third parameter to jx9_context_realloc_chunk().
Calling jx9_context_free_chunk() with a pointer previously returned by jx9_context_alloc_chunk() or jx9_context_realloc_chunk() releases that memory so that it might be reused. Passing a NULL pointer to jx9_context_free_chunk() is harmless. Note that after being freed, memory should neither be read nor written otherwise the result is undefined
Parameters
pCtx |
Foreign function Call Context. |
Return value
A pointer to a freshly allocated/re-allocated chunk is returned on success. NULL is returned on failure (an out-of-memory).
jx9_context_user_data
void * jx9_context_user_data(jx9_context *pCtx);
Extract foreign function private data.
Description
The jx9_context_user_data() interface returns a copy of the pointer that was the pUserData parameter (the last parameter) of the jx9_create_function() that originally registered the application defined function.
This routine accepts as its first argument a pointer to a jx9_context which mean that is designed to be invoked only from a foreign function.
This routine must be called from the same thread in which the application-defined function is running.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
A copy of the pointer untouched.
Stack-able Foreign Function Auxiliary Data
int jx9_context_push_aux_data(jx9_context *pCtx,void *pUserData);
void * jx9_context_peek_aux_data(jx9_context *pCtx);
void * jx9_context_pop_aux_data(jx9_context *pCtx);
Push/Peek/Pop foreign function auxiliary private data.
Description
These interfaces are available to foreign functions in the case they need a stack for an arbitrary number of auxiliary private data perhaps to keep some state information (i.e: the built-in strtok() function rely on these routines heavily).
The jx9_context_push_aux_data() routine pushes an arbitrary pointer in the auxiliary data stack.
The jx9_context_peek_aux_data() routine return the last pushed user pointer in the auxiliary data stack.
The jx9_context_pop_aux_data() pops and returns the last pushed user pointer in the auxiliary data stack.
These interfaces accepts as their first argument a pointer to a jx9_context which mean that they are designed to be invoked only from a foreign function. Also note that each registered foreign function have its own auxiliary data stack.
These routines must be called from the same thread in which the application-defined function is running.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
jx9_context_push_aux_data() return JX9_OK if the pointer was successfully pushed on the stack. Any other return value indicates failure (Out of memory)
jx9_context_peek_aux_data()/jx9_context_pop_aux_data() return the last pushed user pointer in the auxiliary data stack or NULL if the stack is empty.
jx9_context_result_buf_length
unsigned int jx9_context_result_buf_length(jx9_context *pCtx);
Call context result buffer length.
Description
This routine return the size of the call context result buffer. The size of this buffer depends on the number of successive calls to jx9_result_string() or jx9_result_string_format().
This routine accepts as its first argument a pointer to a jx9_context which mean that is designed to be invoked only from a foreign function.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
Call context result buffer length in bytes.
jx9_context_random_num/random_string
unsigned int jx9_context_random_num(jx9_context *pCtx);
int jx9_context_random_string(jx9_context *pCtx,char *zBuf,int nBuflen);
Generate random number/string.
Description
JX9 contains a high-quality pseudo-random number generator (PRNG) based on the one found in the SQLite3 library. The PRNG is also used by the built-in rand() and rand_str() JX9 functions. These interfaces allows host-applications to access the same PRNG for other purposes.
jx9_context_random_num() returns a 32-bit unsigned integer between 0 and 0xFFFFFFFF.
jx9_context_random_string() generate an English based alphabet string of length nBuflen (last argument).
Note that the generated string is not null terminated and the given buffer must be big enough to hold at least 3 bytes.
These interfaces accepts as their first argument a pointer to a jx9_context which mean that they are designed to be invoked only from a foreign function.
These routines must be called from the same thread in which the application-defined function is running.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
Random generated number/string.
jx9_function_name
const char * jx9_function_name(jx9_context *pCtx);
Return the name of the invoked foreign function.
Description
This routine return the name of the invoked foreign function.
This routine accepts as its first argument a pointer to a jx9_context which mean that is designed to be invoked only from a foreign function.
This routine never fail and always return a pointer to a null terminated string.
Parameters
pCtx |
Foreign Function Call Context. |
Return value
A pointer to a null terminated string holding the foreign function name.
Global Library Management Interfaces
int jx9_lib_init(void);
int jx9_lib_config(int nConfigOp,...);
int jx9_lib_shutdown(void);
int jx9_lib_is_threadsafe(void);
const char * jx9_lib_version(void);
const char * jx9_lib_signature(void);
const char * jx9_lib_ident(void);
const char * jx9_lib_copyright(void);
Manual Library Management Interfaces.
Description
The jx9_lib_init() routine initializes the JX9 library. The jx9_lib_shutdown() routine deallocates any resources that were allocated by jx9_lib_init(). These routines are designed to aid in process initialization and shutdown on embedded systems. Workstation applications using JX9 normally do not need to invoke either of these routines.
Note that JX9 is a self initializing library and so a manual call to jx9_lib_init() is not needed. That is, the first call to jx9_init() will automatically initialize the library.
A call to jx9_lib_init() is an "effective" call if it is the first time jx9_lib_init() is invoked during the lifetime of the process, or if it is the first time jx9_lib_init() is invoked following a call to jx9_lib_shutdown(). Only an effective call of jx9_lib_init() does any initialization. All other calls are harmless no-ops.
A call to jx9_lib_shutdown() is an "effective" call if it is the first call to jx9_lib_shutdown() since the last jx9_lib_init() . Only an effective call to jx9_lib_shutdown() does any deinitialization. All other valid calls to jx9_lib_shutdown() are harmless no-ops.
The jx9_lib_init() interface is threadsafe, but jx9_lib_shutdown() is not. The jx9_lib_shutdown() interface must only be called from a single thread. This routine will take care of destroying any open JX9 engines handles and their associated active Virtual Machines.
The jx9_lib_config() interface is used to make global configuration changes to JX9 in order to tune JX9 to the specific needs of the application. The default configuration is recommended for most applications and so this routine is usually not necessary. It is provided to support rare applications with unusual needs.
The jx9_lib_config() interface is not threadsafe. The application must insure that no other JX9 interfaces are invoked by other threads while jx9_lib_config() is running. Furthermore, jx9_lib_config() may only be invoked prior to library initialization using jx9_lib_init() or after shutdown by jx9_lib_shutdown(). If jx9_lib_config() is called after jx9_lib_init() and before jx9_lib_shutdown() then it will return JX9_CORRUPT.
The first argument to jx9_lib_config() is an integer configuration option that determines what property of JX9 is to be configured. Subsequent arguments vary depending on the configuration option in the first argument. Here is the list of allowed verbs
Configuration Verb |
Expected Arguments |
Description |
JX9_LIB_CONFIG_USER_MALLOC |
One Argument: const SyMemMethods *pMethods
|
This option takes a single argument which is a pointer to an instance of the SyMemMethods structure. The argument specifies alternative low-level memory allocation routines to be used in place of the memory allocation routines built into JX9. Note that JX9 will not make a private copy of the content of the SyMemMethods structure which mean that the instance must be available during the life of the library. |
JX9_LIB_CONFIG_MEM_ERR_CALLBACK |
Two Arguments: int (*ProcMemError) (void *) void *pUserData
|
This option takes two arguments: a pointer to a host-application defined function which is invoked by the engine when running out-of-memory. The callback must take one argument which is an arbitrary user pointer. The second argument this option takes is the user pointer which is forwarded verbatim by the engine as the first argument to the callback. If the callback wishes to retry the failed memory allocation process it must return SXERR_RETRY. Note that running out-of-memory is an extremely unlikely scenario on modern hardware even on modern embedded system and so this option is not important as it might looks. |
JX9_LIB_CONFIG_USER_MUTEX |
One Argument: const SyMutexMethods *pMethods |
This option takes a single argument which is a pointer to an instance of the SyMutexMethods structure. The argument specifies alternative low-level mutex routines to be used in place of the mutex routines built into JX9. Note that JX9 will not make a private copy of the content of the SyMutexMethods structure which mean that the instance must be available during the life of the library. |
JX9_LIB_CONFIG_THREAD_LEVEL_SINGLE |
No Arguments |
This option sets the threading mode to Single-thread. In other words, mutexing is disabled and JX9 is put in a mode where it can only be used by a single thread. This option have meaning only if JX9 is compiled with the JX9_ENABLE_THREADS directive enabled. |
JX9_LIB_CONFIG_THREAD_LEVEL_MULTI |
No Arguments |
This option sets the threading mode to Serialized. In other words, all mutexes are enabled including the recursive mutexes on JX9 engine instance and Virtual Machine objects. In this mode (which is the default when JX9 is compiled with JX9_ENABLE_THREADS) the JX9 library will itself serialize access to JX9 engine instance and Virtual Machine so that the application is free to use the same JX9 engine instance or the same Virtual Machine in different threads at the same time. This option have meaning only if JX9 is compiled with the JX9_ENABLE_THREADS directive enabled. |
JX9_LIB_CONFIG_VFS |
One Argument: const jx9_vfs *pVfs |
This option takes a single argument which is a pointer to an instance of the jx9_vfs structure. The argument specifies alternative Virtual File System routines to be used in place of the built-in VFS which is the recommended one for Windows and UNIX systems. Note that JX9 will not make a private copy of the content of the jx9_vfs structure which mean that the instance must be available during the life of the library. |
The jx9_lib_is_threadsafe() function returns TRUE (1) if and only if JX9 was compiled with threading support enabled which is not the default behavior.
JX9 can be compiled with threading support enabled only if the JX9_ENABLE_THREADS compile-time directive is defined. Without multi-threading support (default build) it is not safe to use JX9 concurrently from more than one thread.
Enabling mutexes incurs a measurable performance penalty. So if speed is of utmost importance, it makes sense to disable the mutexes.
jx9_lib_version(), jx9_lib_signature(), jx9_lib_ident() and jx9_lib_copyright() returns a pointer to a null terminated string holding respectively the current version of the JX9 engine, the library signature, the library identification in the symisc source tree and the copyright notice.
Return value
jx9_lib_init(), jx9_lib_shutdown() and jx9_lib_config() returns JX9_OK on success. Any other return value indicates failure.
jx9_lib_version(), jx9_lib_signature(), jx9_lib_ident() and jx9_lib_copyright() never fail and always return a pointer to a null terminated string.
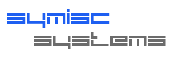