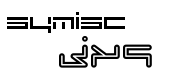
An Embeddable Scripting Engine |
Tweet |
Follow @jx9_engine |
Jx9 C/C++ API Reference - List Of Objects.
This is a list of all abstract objects and datatypes used by the Jx9 library. There are seven objects in total, but the two most important objects are: An engine handle jx9 and a virtual machine object jx9_vm.
|
Jx9 Engine Handle
typedef struct jx9 jx9;
Each active Jx9 engine is represented by a pointer to an instance of the opaque structure named "jx9". It is useful to think of an jx9 pointer as an object. The jx9_init() interface is its constructor and jx9_release() is its destructor. There is also the jx9_config() interface which is used to configure a working jx9 engine and the most important interfaces are the compile interfaces: jx9_compile() and jx9_compile_file(). That is, these interfaces takes as their input a Jx9 program to compile and produce Jx9 bytecode program represented by an opaque pointer to the jx9_vm structure (see below for more information on this structure).
Jx9 Virtual Machine Object
typedef struct jx9_vm jx9_vm;
An instance of this object represents a compiled Jx9 program. this structure hold the Jx9 bytecode program resulting from successful compilation of the target Jx9 script using one of the jx9_compile() or jx9_compile_file() interfaces.
The life of a jx9 virtual machine goes something like this:
-
Compile your Jx9 script using one of the jx9_compile() or jx9_compile_file() interfaces. On successful compilation, the Jx9 engine will automatically create an instance of this structure (jx9_vm) and a pointer to this structure is made available to the caller.
-
When something goes wrong while compiling the Jx9 script due to a compile-time error, the caller must discard this pointer and fix its erroneous Jx9 code. Compile-time error messages can be extracted via a call to jx9_config().
-
Configure the virtual machine using the jx9_vm_config() interface with it's many configuration verbs, but the most important option is set via the JX9_VM_CONFIG_OUTPUT verb which is used to register a VM output consumer callback. That is, an user defined function responsible of consuming the VM output such as redirecting it [i.e: the VM output] to the standard output (STDOUT) or sending it back to the connected peer and so on.
-
Optionally, register one or more foreign functions or constants using the jx9_create_function() or jx9_create_constant() interfaces.
-
Execute the compiled Jx9 program by calling jx9_vm_exec().
-
Optionally, extract the contents of one or more variables declared inside your Jx9 script using the jx9_vm_extract_variable() interface.
-
Reset the virtual machine using jx9_vm_reset() then go back to step 5. Do this zero or more times.
-
Destroy the virtual machine using jx9_vm_release().
Dynamically Typed Value Object
typedef struct jx9_value jx9_value;
Jx9 uses the jx9_value object to represent all values that can be stored in a Jx9 variable. Since Jx9 uses dynamic typing for the values it stores. Values stored in jx9_value objects can be integers, floating point values, strings, JSON arrays, JSON objects and so forth.
Internally, the Jx9 virtual machine manipulates nearly all Jx9 values as jx9_values structures. Each jx9_value may cache multiple representations (string, integer, etc.) of the same value.
The
jx9_value object is heavily used in the interaction between the Jx9
virtual machine and the host application especially in the
implementation of application-defined
foreign functions and/or
constant expansion
callbacks.
There are a dozens of interfaces that allow the host application to extract and manipulates jx9_value cleanly, these includes:
jx9_value_to_int(), jx9_value_to_string(), jx9_value_compare(), jx9_value_int64(), jx9_value_bool(), jx9_value_is_null(), jx9_value_is_float(), jx9_value_is_callable(), jx9_value_string_format() and many more. Refer to the C/C++ Interfaces documentation for additional information.
Foreign Function Context Object
typedef struct jx9_context jx9_context;
The context in which a foreign function executes is stored in a jx9_context object. A pointer to a jx9_context object is always first parameter to application-defined foreign functions. The application-defined foreign function implementation will pass this pointer through into calls to dozens of interfaces, these includes jx9_result_int(), jx9_result_string(), jx9_result_value(), jx9_context_new_scalar(), jx9_context_alloc_chunk(), jx9_context_output(), jx9_context_throw_error() and many more. Refer to the C/C++ Interfaces documentation for additional information.
You can refer to the following guide for an introductory course to this interface and the foreign function mechanism in general.
64-Bit Integer Types
#if defined(_MSC_VER) || defined(__BORLANDC__)
typedef signed __int64 jx9_int64;
#else
typedef signed long long int jx9_int64;
#endif /* _MSC_VER */
Jx9 uses 64-bit integer for integers arithmetic regardless of the target platform. Because there is no cross-platform way to specify 64-bit integer types Jx9 includes typedefs for 64-bit signed integers. The jx9_int64 type can store integer values between -9223372036854775808 and +9223372036854775807 inclusive. Some of the interface that manipulates 64-bit integers are jx9_value_int64(), jx9_result_int64(), jx9_value_to_int64().
Jx9 IO Stream
typedef struct jx9_io_stream jx9_io_stream;
struct jx9_io_stream
{
const char *zName; /* Underlying stream name [i.e: file/http/zip/jx9,..] */
int iVersion; /* IO stream structure version [default 1]*/
int (*xOpen)(const char *,int,jx9_value *,void **); /* Open handle*/
int (*xOpenDir)(const char *,jx9_value *,void **); /* Open directory handle */
void (*xClose)(void *); /* Close file handle */
void (*xCloseDir)(void *); /* Close directory handle */
jx9_int64 (*xRead)(void *,void *,jx9_int64); /* Read from the open stream */
int (*xReadDir)(void *,jx9_context *); /* Read entry from directory handle */
jx9_int64 (*xWrite)(void *,const void *,jx9_int64); /* Write to the open stream */
int (*xSeek)(void *,jx9_int64,int); /* Seek on the open stream */
int (*xLock)(void *,int); /* Lock/Unlock the open stream */
void (*xRewindDir)(void *); /* Rewind directory handle */
jx9_int64 (*xTell)(void *); /* Current position of the stream read/write pointer */
int (*xTrunc)(void *,jx9_int64); /* Truncates the open stream to a given length */
int (*xSync)(void *); /* Flush open stream data */
int (*xStat)(void *,jx9_value *,jx9_value *); /* Stat an open stream handle */
};
An instance of the jx9_io_stream object defines the interface between the Jx9 core and the underlying stream device.
A stream is a smart mechanism for generalizing file, network, data compression and other IO operations which share a common set of functions using an abstracted unified interface.
A stream device is additional code which tells the stream how to handle specific protocols/encodings. For example, the http device knows how to translate a URL into an HTTP/1.1 request for a file on a remote server. Jx9 come with two built-in IO streams device. The file:// stream which perform very efficient disk IO and the jx9:// stream which is a special stream that allow access various I/O streams.
A stream is referenced as: scheme://target
-
scheme(string) - The name of the wrapper to be used. Examples include: file, http, https, ftp and jx9. If no wrapper is specified, the function default is used (typically file://).
-
target - Depends on the wrapper used. For filesystem related streams this is typically a path and filename of the desired file. For network related streams this is typically a hostname,often with a path appended.
IO stream devices are registered using a call to jx9_vm_config() with a configuration verb set to JX9_VM_CONFIG_IO_STREAM.
Currently the Jx9 development team is working on the implementation of the http:// and ftp:// IO stream protocols. These devices will be available in the next release of Jx9.
Developers wishing to implement their own IO stream devices must understand and follow The JX9 IO Stream C/C++ Specification Manual.
Jx9 Virtual File Systems (VFS)
typedef struct jx9_vfs jx9_vfs;
struct jx9_vfs
{
const char *zName; /* Underlying VFS name [i.e: FreeBSD/Linux/Windows...] */
int iVersion; /* Current VFS structure version [default 2] */
/* Directory functions */
int (*xChdir)(const char *); /* Change directory */
int (*xChroot)(const char *); /* Change the root directory */
int (*xGetcwd)(jx9_context *); /* Get the current working directory */
int (*xMkdir)(const char *,int,int); /* Make directory */
int (*xRmdir)(const char *); /* Remove directory */
int (*xIsdir)(const char *); /* Tells whether the filename is a directory */
int (*xRename)(const char *,const char *); /* Renames a file or directory */
int (*xRealpath)(const char *,jx9_context *); /* Return canonicalized absolute pathname*/
/* Systems functions */
int (*xSleep)(unsigned int); /* Delay execution in microseconds */
int (*xUnlink)(const char *); /* Deletes a file */
int (*xFileExists)(const char *); /* Checks whether a file or directory exists */
int (*xChmod)(const char *,int); /* Changes file mode */
int (*xChown)(const char *,const char *); /* Changes file owner */
int (*xChgrp)(const char *,const char *); /* Changes file group */
jx9_int64 (*xFreeSpace)(const char *); /* Available space on filesystem or disk partition */
jx9_int64 (*xTotalSpace)(const char *); /* Total space on filesystem or disk partition */
jx9_int64 (*xFileSize)(const char *); /* Gets file size */
jx9_int64 (*xFileAtime)(const char *); /* Gets last access time of file */
jx9_int64 (*xFileMtime)(const char *); /* Gets file modification time */
jx9_int64 (*xFileCtime)(const char *); /* Gets inode change time of file */
int (*xStat)(const char *,jx9_value *,jx9_value *); /* Gives information about a file */
int (*xlStat)(const char *,jx9_value *,jx9_value *); /* Gives information about a file */
int (*xIsfile)(const char *); /* Tells whether the filename is a regular file */
int (*xIslink)(const char *); /* Tells whether the filename is a symbolic link */
int (*xReadable)(const char *); /* Tells whether a file exists and is readable */
int (*xWritable)(const char *); /* Tells whether the filename is writable */
int (*xExecutable)(const char *); /* Tells whether the filename is executable */
int (*xFiletype)(const char *,jx9_context *); /* Gets file type [i.e: fifo,dir,file..] */
int (*xGetenv)(const char *,jx9_context *); /* Gets the value of an environment variable */
int (*xSetenv)(const char *,const char *); /* Sets the value of an environment
variable */
int (*xTouch)(const char *,jx9_int64,jx9_int64); /* Sets access and modification time
* of file */
int (*xMmap)(const char *,void **,jx9_int64 *); /* Read-only memory map of the whole file */
void (*xUnmap)(void *,jx9_int64); /* Unmap a memory view */
int (*xLink)(const char *,const char *,int); /* Create hard or symbolic link */
int (*xUmask)(int); /* Change the current umask */
void (*xTempDir)(jx9_context *); /* Get path of the temporary directory */
unsigned int (*xProcessId)(void); /* Get running process ID */
int (*xUid)(void); /* user ID of the process */
int (*xGid)(void); /* group ID of the process */
void (*xUsername)(jx9_context *); /* Running username */
int (*xExec)(const char *,jx9_context *); /* Execute an external program */
};
An instance of the jx9_vfs object defines the interface between the Jx9 core and the underlying operating system. The "vfs" in the name of the object stands for "virtual file system". The vfs is used to implement Jx9 system functions such as mkdir(), chdir(), stat() and many more.
The value of the iVersion field is initially 2 but may be larger in future versions of Jx9. Additional fields may be appended to this object when the iVersion value is increased.
Only a single vfs can be registered within the Jx9 core. Vfs registration is done using the jx9_lib_config() interface with a configuration verb set to JX9_LIB_CONFIG_VFS.
Note that Windows and UNIX (Linux, FreeBSD, Solaris, MacOS X, etc.) users does not have to worry about registering and installing their own vfs, now Jx9 come with a built-in vfs that implements most the methods defined above.
Host applications running on exotic systems (ie: Other than Windows and UNIX systems) must register their own vfs in order to be able to use and call Jx9 system function. Also note that the jx9_compile_file() interface depends on the xMmap() method of the underlying vfs which mean that this method must be available (Always the case using the built-in VFS) in order to use this interface.
Developers wishing to implement their own vfs must contact Symisc Systems inorder to obtain the JX9 VFS C/C++ Specification Manual.
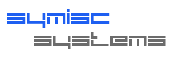