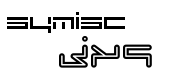
An Embeddable Scripting Engine |
Tweet |
Follow @jx9_engine |
Foreign Function Implementation Guide.
The following quick guide is what you do to start experimenting with the JX9 foreign function mechanism without having to do a lot of tedious reading.
Foreign functions are used to add JX9 functions or to redefine the behavior of existing JX9 functions from the outside environment (see below) to the underlying virtual machine. This mechanism is know as “In-process extending”. After successful call to jx9_create_function(), the installed function is available immediately and can be called from the target JX9 code.
A foreign function is simply a host application defined function typically implemented in C which is responsible of performing the desired computation. There are many reasons to implement foreign functions, one of them is to perform system calls and gain access to low level resources that is impossible to do using standard JX9 functions.
Another reason to implement foreign functions is performance. That is, a foreign function implemented in C will typically run 5 times faster than if it was implemented in JX9, this is why most of the built-in JX9 functions (Over 303 as of this release) such as json_encode(), crc32(), array_merge(), chdir(), fread(), fopen() and many more are implemented in C rather than JX9.
The signature of the foreign function is as follows:
int (*xFunc)(jx9_context *pCtx,int argc,jx9_value **argv)
As you can see, the implemented function must accept three parameters. The first parameter is a pointer to a jx9_context structure. This is the context in which the foreign function executes. In other words, this parameter is the intermediate between the foreign function and the underlying virtual machine.
The application-defined foreign function implementation will pass this pointer through into calls to dozens of interfaces, these includes:
jx9_context_output(), jx9_context_throw_error(), jx9_context_new_scalar(), jx9_context_user_data(), jx9_context_alloc_chunk() and many more.
The second parameter is the total number of arguments passed to the foreign function. If the total number of arguments is not the expected one, the foreign functions can throw an error via jx9_context_throw_error() as follow: jx9_context_throw_error(pCtx,JX9_CTX_WARNING,"Unexpected number of arguments");
The last parameter is an array of pointers to jx9_value which represents function arguments. The implementation of the foreign functions can extract their contents via one of these interfaces:
The computation result (i.e. the return value) of the foreign function can be set via one of these interfaces:
If no call is made to one of these interfaces, then a NULL return value is assumed.
The implementation of the foreign function must return JX9_OK on success, but if the callbacks wishes to abort processing (to stop program execution) and thus to emulate the exit or die JX9 constructs, it must return JX9_ABORT instead.
Typical
Implementation Of A Foreign Function
A typical implementation of a foreign function would perform the following operations in order:
-
Check if the given arguments are of the expected numbers and expected types. If the foreign accepts arguments, this is the first operation it must perform before doing any serious work. For that, a set of interfaces are available:
To check if the given arguments are of the expected number, simply compare the expected number with the argc parameter (Second parameter the foreign function takes).
-
If the foreign function accepts arguments, it may need to extract their contents via the following set of interfaces:
-
Perform the desired computation.
-
Allocate additional jx9_value via the jx9_context_new_scalar() and/or jx9_context_new_array() interfaces especially if the foreign function works with JSON arrays or JSON objects (see below for a working example).
-
If something goes wrong while processing the input or performing the computation, the foreign function can throw an error via the jx9_context_throw_error() or jx9_context_throw_error_format() interfaces.
-
If the foreign function wishes to output a message and thus to emulate the print JX9 construct, it may call jx9_context_output() or jx9_context_output_format().
-
When done, the computation result (i.e. return value) of the foreign function can be set via one of the these interfaces:
-
And finally, return JX9_OK when done. Note that you do not need to release any allocated resource manually via the jx9_context_release_value() interface, the virtual machine will release any allocated resources for you automatically.
Foreign Function Examples
We will start our examples with the simplest foreign function implemented in C which perform a simple right shift operation on a given number and return the new shifted value as it's computation result (i.e. return value), here is the C code:
-
int shift_func(
-
jx9_context *pCtx, /* Call Context */
-
int argc, /* Total number of arguments passed to the function */
-
jx9_value **argv /* Array of function arguments */
-
)
-
{
-
int num;
-
/* Make sure there is at least one argument and is of the
-
* expected type [i.e: numeric].
-
*/
-
if( argc < 1 || !jx9_value_is_numeric(argv[0]) ){
-
/*
-
* Missing/Invalid argument,throw a warning and return FALSE.
-
* Note that you do not need to log the function name,JX9 will
-
* automatically append the function name for you.
-
*/
-
jx9_context_throw_error(pCtx,JX9_CTX_WARNING,"Missing numeric argument");
-
/* Return false */
-
jx9_result_bool(pCtx,0);
-
return JX9_OK;
-
}
-
/* Extract the number */
-
num = jx9_value_to_int(argv[0]);
-
/* Shift by 1 */
-
num <<= 1;
-
/* Return the new value */
-
jx9_result_int(pCtx,num);
-
/* All done */
-
return JX9_OK;
-
}
On line 11, we check if there is at least one argument and is numeric (integer or float or a string that looks like a number). If not, we throw an error on line 17 and we return a Boolean false (zero) on line 19 using the jx9_result_bool() interfaces.
We extract the function argument which is the number to shift on line 23 using the jx9_value_to_int() interface.
We
perform the desired computation (right shift the given number by one)
on line 25.
And finally, we return the new value as our computation result one line 27 using a call to jx9_result_int().
Now after installing this function (see below for a working example), it can be called from your JX9 script as follows:
print shift_func(150); //you should see 300.
Another simple foreign function named date_func(). This function does not expect arguments and return the current system date in a string. A typical call to this function would return something like: 2012-23-09 13:53:30. Here is the implementation
-
int date_func(
-
jx9_context *pCtx, /* Call Context */
-
int argc, /* Total number of arguments passed to the function */
-
jx9_value **argv /* Array of function arguments*/
-
){
-
time_t tt;
-
struct tm *pNow;
-
/* Get the current time */
-
time(&tt);
-
pNow = localtime(&tt);
-
/*
-
* Return the current date.
-
*/
-
jx9_result_string_format(pCtx,
-
"%04d-%02d-%02d %02d:%02d:%02d", /* printf() style format */
-
pNow->tm_year + 1900, /* Year */
-
pNow->tm_mday, /* Day of the month */
-
pNow->tm_mon + 1, /* Month number */
-
pNow->tm_hour, /* Hour */
-
pNow->tm_min, /* Minutes */
-
pNow->tm_sec /* Seconds */
-
);
-
/* All done */
-
return JX9_OK;
-
}
We get the date on line 10 using the libc localtime() routine and we return it using the jx9_result_string_format() interface on 14. This function is a work-alike of the "printf()" family of functions from the standard C library which is used to append a formatted string.
Download & Compile this C file for a working version of the function defined above plus others foreign functions.
Working
With JSON Arrays/Object
Working with JSON arrays or objects is relatively easy and involves two or three additional call to JX9 interfaces. A typical implementation of a foreign function that works with arrays/objects would perform the following operations:
-
Call jx9_context_new_array() to allocate a fresh jx9_value of type array or object. The new array/object is empty and need to be populated. This interface is unified for JSON arrays as well JSON objects.
-
Call jx9_context_new_scalar() to allocate a new scalar jx9_value. This value is used to populate array/object entries with the desired value.
-
Populate the array/object using one or more calls to jx9_array_add_elem() and/or it's wrappers. Again, these interfaces are unified for JSON arrays and objects.
-
Finally, return the fresh array/object using the jx9_result_value() interface.
We will start our examples by a simple foreign function names array_time_func(). This function does not expects arguments and return the current time in a JSON array. A typical output of this function would look like this:
/* JSON Array */
[14,53,30]
Here is the C code.
int array_time_func(jx9_context *pCtx,int argc,jx9_value **argv)
{
jx9_value *pArray; /* Our JSON Array */
jx9_value *pValue; /* Array entries value */
time_t tt;
struct tm *pNow;
/* Get the current time first */
time(&tt);
pNow = localtime(&tt);
/* Create a new json array */
pArray = jx9_context_new_array(pCtx);
/* Create a worker scalar value */
pValue = jx9_context_new_scalar(pCtx);
if( pArray == 0 || pValue == 0 ){
/*
* If the supplied memory subsystem is so sick that we are unable
* to allocate a tiny chunk of memory, there is no much we can do here.
* Abort immediately.
*/
jx9_context_throw_error(pCtx,JX9_CTX_ERR,"Fatal, JX9 is running out of memory");
/* emulate the die() construct */
return JX9_ABORT; /* die('Fatal,JX9 is running out of memory'); */
}
/* Populate the JSON array.
* Note that we will use the same worker scalar value (pValue) here rather than
* allocating a new value for each array entry. This is due to the fact
* that the populated array will make it's own private copy of the inserted
* key(if available) and it's associated value.
*/
jx9_value_int(pValue,pNow->tm_hour); /* Hour */
/* Insert the hour at the first available index */
jx9_array_add_elem(pArray,0/* NULL: Assign an automatic index*/,pValue /* Will make it's own copy */);
-
/* Overwrite the previous value */
jx9_value_int(pValue,pNow->tm_min); /* Minutes */
/* Insert minutes */
jx9_array_add_elem(pArray,0/* NULL: Assign an automatic index*/,pValue /* Will make it's own copy */);
-
/* Overwrite the previous value */
jx9_value_int(pValue,pNow->tm_sec); /* Seconds */
/* Insert seconds */
jx9_array_add_elem(pArray,0/* NULL: Assign an automatic index*/,pValue /* Will make it's own copy */);
-
/* Return the array as the function return value */
jx9_result_value(pCtx,pArray);
-
/* All done. Don't worry about freeing memory here, every
* allocated resource will be released automatically by the engine
* as soon we return from this foreign function.
*/
return JX9_OK;
-
}
We allocate a fresh empty JSON array on line 11 using a call to jx9_context_new_array(). Also, we allocate a fresh scalar value one line 13 using a call to jx9_context_new_scalar().
We populate the scalar jx9_value with the desired value, here the current hour on line 31 using a call to jx9_value_int() and we insert it in the new array using the jx9_array_add_elem() interface on line 33.
This process is repeated again on line 36, 38, 41 and 43. Note that we use the same scalar value to populate the array with the three entries. This is due to the fact that a call to jx9_array_add_elem() and it's wrappers will make a private copy of the inserted value and so it's safe to use the same scalar value for other insertions.
Finally, we return our array as our computation result one line 46 using the jx9_result_value() interface.
Test The Implementation
Now we
have implemented our foreign functions, it's time to test them. For
that, we will create a simple JX9 program that call each of the
defined functions and output their return values. Here is the program:
print 'shift_func(150) = ' .. shift_func(150) .. JX9_EOL;
print 'sum_func(7,8,9,10) = ' .. sum_func(7,8,9,10) .. JX9_EOL;
print 'date_func(5) = ' .. date_func() .. JX9_EOL;
print 'array_time_func() =' .. array_time_func() .. JX9_EOL;
print 'object_date_func() =' .. JX9_EOL;
dump(object_date_func());
print 'array_str_split(\'Hello\') =' .. JX9_EOL;
dump(array_str_split('Hello'));
When running this program, you should see something like that:
shift_func(150) = 300
sum_func(7,8,9,10) = 34
date_func(5) = 2012-29-12 01:13:58
array_time_func() =[1,13,58]
object_date_func() =
JSON Object(6 {
"tm_year":2012,
"tm_mon":12,
"tm_mday":29,
"tm_hour":1,
"tm_min":13,
"tm_sec":58
}
)
array_str_split('Hello') =
JSON Array(5 ["H","e","l","l","o"])
Now, the main program.(You can get a working version of this program here)
/*
* Container for the foreign functions defined above.
* These functions will be registered later using a call
* to [jx9_create_function()].
*/
static const struct foreign_func {
const char *zName; /* Name of the foreign function*/
int (*xProc)(jx9_context *,int,jx9_value **); /* Pointer to the C function performing the computation*/
}aFunc[] = {
{"shift_func",shift_func},
{"date_func", date_func},
{"sum_func", sum_func },
{"array_time_func", array_time_func},
{"array_str_split", array_string_split_func},
{"object_date_func", object_date_func}
};
/*
* Main program: Register the foreign functions defined above, compile and execute
* our JX9 test program.
*/
int main(void)
{
jx9 *pEngine; /* JX9 engine */
jx9_vm *pVm; /* Compiled JX9 program */
int rc;
int i;
/* Allocate a new JX9 engine instance */
rc = jx9_init(&pEngine);
if( rc != JX9_OK ){
-
Fatal("Error while allocating a new JX9 engine instance");
}
/* Compile the JX9 test program defined above */
rc = jx9_compile(
pEngine, /* JX9 engine */
JX9_PROG, /* JX9 test program */
-1 /* Compute input length automatically*/,
&pVm /* OUT: Compiled JX9 program */
);
if( rc != JX9_OK ){
if( rc == JX9_COMPILE_ERR ){
const char *zErrLog;
int nLen;
/* Extract error log */
jx9_config(pEngine,
&zErrLog,
&nLen
);
if( nLen > 0 ){
/* zErrLog is null terminated */
puts(zErrLog);
}
}
/* Exit */
Fatal("Compile error");
}
/* Now we have our program compiled,it's time to register
* our foreign functions.
*/
for( i = 0 ; i < (int)sizeof(aFunc)/sizeof(aFunc[0]) ; ++i ){
/* Install the foreign function */
rc = jx9_create_function(pVm,aFunc[i].zName,aFunc[i].xProc,0 /* NULL: No private data */);
if( rc != JX9_OK ){
Fatal("Error while registering foreign functions");
}
}
-
/*
* Configure our VM:
* Install the VM output consumer callback defined above.
*/
rc = jx9_vm_config(pVm,
Output_Consumer, /* Output Consumer callback */
0 /* Callback private data */
);
if( rc != JX9_OK ){
Fatal("Error while installing the VM output consumer callback");
}
-
/*
* Report run-time errors such as unexpected numbers of arguments and so on.
*/
-
/*
* And finally, execute our program. Note that your output (STDOUT in our case)
* should display the result.
*/
jx9_vm_exec(pVm,0);
-
/* All done, cleanup the mess left behind.
*/
jx9_vm_release(pVm);
jx9_release(pEngine);
-
return 0;
}
We create a new JX9 engine instance using a call to jx9_init() on line 29. This is often the first JX9 API call that an application makes and is a prerequisite in order to compile JX9 code using one of the compile interfaces.
We compile our JX9 test program on line 35 using the jx9_compile() interface.
We register our implemented foreign functions on line 66 using the jx9_create_function() interface.
We configure our Virtual Machine on line 77 by setting a VM output consumer callback named Output_Consumer() (Download the C file to see the implementation) which redirect the VM output to STDOUT.
And finally we execute our JX9 program on line 97 using a call to jx9_vm_exec().
Clean-up is done on line 101 and 102 respectively via calls to jx9_vm_release() and jx9_release().
Other useful links
As you can see, in-process extending under JX9 is extremely powerful yet simple and involves only a single call to jx9_create_function().
Check out the Introduction To The JX9 C/C++ Interface for an introductory overview and roadmap to the dozens of JX9 interface functions.
A separate document, The JX9 C/C++ Interface, provides detailed specifications for all of the various C/C++ APIs for JX9. Once the reader understands the basic principles of operation for JX9, that document should be used as a reference guide.
Any questions, check the Frequently Asked Questions page or visit the Support Page for additional information.
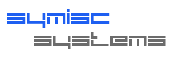